Applet Output
Prerequisite: Running Java Programs Lesson, Chapter 1
At the end of this you should be able to:
1. Explain the parts of a Java applet and how it works
2. Use the drawstring method to format output
3. Explain Java built-in classes and browse through methods
4. Change the size and properties of text using the Font class
5. Create an applet with GUI components
6. Use Graphic objects and methods in an applet
7. Compare Java 1.1 and 1.2 applets
8. Use Image elements in an applet
9. Use a simple MouseEvent applet
10. Produce a Java applet with the above Graphic elements
Open a Java project called AppletOutput. Select New in Jbuilder and double-click on the Applet icon. Erase the Package text and call your class ApOut. Delete the default java template code. Your screen should look like this:
Now, lets cut and paste (or load from your floppy) the program below that we have seen before.
import java.applet.Applet; import java.awt.*; public class ApOut extends Applet { public void paint (Graphics g) {g.drawString("Hello world",10,10);} }
Make sure you can run this program, as you did in the Running Java Program lesson. Let’s see what happens in this program. The first 2 lines of the program: import java.applet.Applet; import java.awt.*; Serve the purpose of telling Jbuilder, or the Java compiler you are using, that your program uses features in the Java language that are not available by default. These 2 lines say that the following program uses classes in the applet package and the awt package. A package is a group of related classes.
So.. a package (such as applet and awt) is like a folder of classes.. but what is a class? A class is like a program template. Think of it as the design of a car. In particular, lets say a Ford Echo. Once you have the design (called a class in Java) you can make as many instances of the car as you want. In Java we call an instance of a class an Object, thus the name Object Oriented Programming. Each instance of the car has the metal, glass, etc that makes up the car. In Java, we call that data. There is also a manufacturing process for the car. In Java we call that the constructor. The car, once made, is able to DO many tasks, such as start, go, go faster, stop, turn on lights, etc.. In Java we call the things that an Object can do its methods. Below is a diagram of a class:
Once we have a class, we can make (or construct) an Object of that type by calling its constructor. Then to get the Object to do something, we call one of its methods. More on this later.
Java is made up of the keywords of the language, described in your text, and a bunch of pre-made classes you can use. Whenever you write a Java program, you get to use all the classes defined in the package java.lang. Notice we did not need to import these classes. Some Java programs, however, will need more than the java.lang classes. We need the Java classes for making Windows components (the awt package). We will be making an Applet and will need the applet classes, defined in the java.applet package. If our program, for example, was not an applet we would not need to import the applet package. This makes our program compile more efficiently, since we only import what we need. Try commenting out one of the import statements and look at the error message. The statement import java.awt.* says to make ALL the classes in the awt package available when the program is compiled into bytecodes. The statement import java.applet.Applet says to import only the Applet class from the package applet. We could have used import java.applet.* and everything world have worked fine. We were just being a little more specific and therefore a bit more efficient.
Now let’s look at the line: public class ApOut extends Applet The word public tells the computer that this class (you can think of it as a program) you are making will be made available to other classes that want to use it. Every class you execute like a program must have one public class. The keyword ‘class’ follows. Notice it is in blue type. This lets you know it is a Java keyword. You can see the others Java keywords as well. Notice ApOut is not in blue. It is YOUR name for this class. It must match with the name of the .java file you make in Jbuilder. The keyword extends tells Java that your class is an example, or instance of another class already made, in this case the Applet class that is defined in the Java language. We say the ApOut class inherits from the Applet class, meaning it will have all the data, constructors, and methods of the Applet class as well as the new ones we define in the ApOut code.
Going back to the car analogy, we can make a Ford Echo convertible by saying that it IS a Ford Echo with the following alterations- then list the alterations. This lets us START with the Echo design and only worry about the alterations. This is what is happening to our Applet. ApOut IS an Applet with the features we will describe between the opening { and the ending }.
Between the {} for the class ApOut we have the following code: public void paint (Graphics g) {g.drawString("Hello world",10,10);}
This code is defining a Method. ApOut does NOT have data or a constructor. We will look at these parts in later classes. A Method tells the object to DO something. In this case, paint is a method ALWAYS called in an Applet that will ‘paint’ something to the browser window. The keyword public, again, means that this method can be seen and used by other classes. Void means that the method does not return a value (more later..) paint, again, is the name of the class. In between the ( ) is what is called the parameter list. In this case, we have the text Graphics g. This means that the paint method, on order to do its job, will need ‘Graphics g’ as input. Think of this as if I told you to Add something. Although you know how to DO addition, you could not proceed with the process until I gave you 2 numbers. These 2 numbers would be the parameters for Add just as Graphics g is the parameter for paint.
Graphics g can be read as the following: Make an object called g out of the class called Graphics. Graphics is a pre-defined class in Java. Let’s explore it now. Do the following:
1. Go to the project AppletOutput and look at your .java code for ApOut. Find Graphics g in your code and click anywhere on the word Graphics. You will get a blinking I-beam cursor.
2. Press the F1 key (help). You will see the following:
3. This is the Jbuilder Help system. It is discussed in your book and you should get familiar with it ASAP. Since we were over Graphics when we pressed F1, it found this class in Java and we can look at the details of this class. First, notice that it is in the package awt, which we imported earlier in our program. Also notice that below class Graphics there is a link to java.Object. This is telling you that like our Applet that extended the class Applet, the class Graphic extends the class Object. You can read about the class Object by clicking on the blue link. The Graphic class will get, or inherit, all the data, constructors, and methods from the Object class. All the classes in Java inherit from the Object class. It is the Base class of Java.
4. Scroll down on the help page. You will see one Constructor for Graphics and many Methods listed. Find the drawString method:
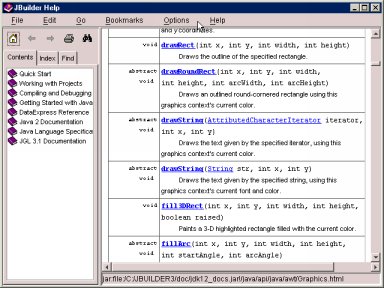 Notice there are MANY other methods for Graphics. You can read about the drawString method. Notice that there are 2 drawString methods. Java knows which one to use based on the parameters. We are giving our drawstring a string and 2 integers for parameters. This will call the second of the drawString methods. The new effect will be to put the given string on the browser at the x,y location given by the integers.
5. Why g? No reason at all. The g is an Object of class Graphics. We could have picked a different letter or identifier. Change paint to this: public void paint (Graphics myPic) {myPic.drawString("Hello world",10,10);} The object myPic in this case is the name of the Object that is of class Graphics. myPic.drawstring(…) is telling the myPic object to call its method drawString. This is called sending a ‘message’ to the object. We can name the object anything we want. The message will be the name of the method we want to use and the correct parameters.
6. Finally, look at the capitalization. A Class name is, by convention, capitalized (Graphics, Applet, ApOut). An object is not capitalized (g, myPic). Methods are not capitalized. This is just to help us. Java could care less. It would have worked fine if we had called our class apOut (as long as the name of our .java file was apOut).
Cut and paste the following code in the ApOut.java file:
import java.applet.Applet; import java.awt.*; public class ApOut extends Applet { public void paint (Graphics g) { g.drawString(" A",10,10); g.drawString(" A A",10,20); g.drawString(" A A",10,30); } }
See if you can figure out how to print your first and last initials in Block letters to the screen by adapting this program. This code is the start of the block A. If you save your program as BlockInits.java, for example, you will have a problem. Now the name of the file (BlockInits) will not match the name of the class (ApOut). You can, however, copy the text of your code and paste it into notepad. Save it there under any name you like. Now you can open, cut and paste your code from your C drive just as you are doing from this website.
Changing the Size and Color of Text.
** When you change your code and re-run a program, sometimes it will still run your old code. To force the ‘new’ program to run by stopping the old program, hold the control key and press F5 when you are in the browser. This will stop the old .class file from running and reload the applet.
Copy and Paste the following program in the ApOut.java content pane. Make sure you do a SaveAs if you want to keep the current contents of the content page in Jbuilder:
import java.applet.Applet; import java.awt.*;
public class ApOut extends Applet { Font font; public void init() { font = new Font("TIMES",font.BOLD,20); } public void paint (Graphics g) { g.setFont(font);g.drawString("Make this big",20,20); g.setColor(Color.red); g.drawString("Colored text!",50,50); } }
In your book you read about the anatomy of an applet. It calls the methods init, start, and paint in that order. If one of these methods is missing, it is skipped. In this program, we have 2 methods and a piece of data. Font font; is data for our class. It can be read as: Make a new object with the name of font from the class called Font. (We could have used Font f , or Font myFont..) Click on Font in Jbuilder and read about the class Font by pressing the F1 key. In the init method we are calling the constructor for class Font and telling our class what kind of Font we want. The paint method can then use the font. Why did we not need to make our new Graphics object for the last program in init? Well, Java- fortunately or unfortunately, does some things for us. We got ‘lucky’ in the last program because Graphics was what is called an ‘abstract’ class that does not need a specific call to its constructor.
Tweak this program so that you have different size, font type, and color for text. Add new text. Experiment!!
Other Graphics methods:
Copy the following program into ApOut:
import java.applet.Applet;
import java.awt.*;
public class ApOut extends Applet
{
public void init()
{
setBackground(Color.pink);
}
public void paint (Graphics g)
{
g.drawLine(5,5,15,300);
g.setColor(Color.cyan);
g.fill3DRect(50,50,30,40,true);
g.drawRect(150,150,30,60);
}
}
Here we are playing with the methods of class Graphics. Notice the 2 types of boxes, the line and the color. Why is the line black? Notice that the line goes off the browser page. Can you fix this? Go to Jbuilder and click on the file ApOut.html. In the bottom left corner of the content pane, find the 2 tabs for View and Source. Click on Source. Make sure you have the ApOut.html file selected. Notice the default size of the Applet is 400 for the width and 300 for the height. Change these values to 600 and 500. Save the file and re-run the program. Notice the size of the applet now.
Go back to ApOut.java. We will enter a new line in our program. Click after the ; in the last line of the program (the g.drawRect line). Hit Enter. Now type ‘g.’ and wait for a second. After a second or two you will see a list of all the methods for an object of class type Graphics. In this pop-up list, scroll down until you see the method called drawOval. Double-click on it. Notice it wades you through what parameters the drawOval method needs. Pick 100,50,20,30. Run your new program. Make sure you enter ; after the line Experiment with other Graphics methods.
GUI components:
Copy the following program into ApOut:
import java.applet.Applet;
import java.awt.*;
public class ApOut extends Applet
{
Label lab1,lab2;
Button b1;
TextField t1;
public void init()
{
lab1 = new Label ("This is label 1");
add(lab1);
lab2 = new Label("This is label 2");
add(lab2);
b1 = new Button("Stop");
add(b1);
t1 = new TextField("enter text here");
add(t1);
}
public void paint(Graphics g)
{
g.drawLine(50,50,100,100);
}
}
Notice this class has 4 pieces of data ( lab1, lab2, b1 and t1). It has 2 methods (init and paint). Lab1 and lab2 are objects of class Label. Click on Label in your code in Jbuilder and press F1 to find out about the Label class. You also have a Button and a TextField object (b1 and t1) In the init method, we call the constructor for the classes we need. Notice that we do NOT tell where to put the objects on the screen as we did with the drawstring method in Graphics. This is because the Applet will automatically put the objects where there is room for them. You can test this out by changing the size of the html screen as we did above. What if you WANTED to put the buttons in a certain place on the screen? You can, but it is not easy. We will find out how to do this task later. For now, live with it.. Try adding more Buttons or Labels to your applet. Are there more GUI classes? Sure! Click on Label in your code and press F1. Notice that Label is not only extended from the Object class, but also the Component class. Click on the Component class link in help. You can find here a list of the known subclasses of Component, such as Button, Label, Choice, Canvas, and several others. Where is TextField? It happens to be a subclass of the TextComponent class on the list. Try adding a Choice object to your applet. How about List? How do you call the constructors? See if you can figure it out from the code above. You can also go to the, say, Choice class help (F1) and scrolling down until you see the constructors to find what parameters they need. Notice that there are several constructors. Your parameters will determine which ones will be used. Again, experiment.
Enter the following code into ApOut:
import javax.swing.*;
import java.awt.*;
public class ApOut extends JApplet
{
JTextField t1;
JButton b1;
JLabel l1;
public void init()
{
Container contentPane = getContentPane();
contentPane.setLayout(new FlowLayout());
contentPane.setBackground(Color.green);
t1 = new JTextField(10);
contentPane.add(t1);
b1 = new JButton("press me");
contentPane.add(b1);
l1 = new JLabel("this is a label");
contentPane.add(l1);
}
}
This is a Java2 or swing applet. Notice it has slightly different imports. It extends Japplet, not Applet. Instead of Button, for instance, it has Jbutton. It is also slightly more complicated with a ContentPane class needed. Also not it works. Java2 is newer. It extends the old java Applet and awt classes and adds some new features. Why did we still mess with the ‘old’ stuff? Go to Windows Explorer and find the Jbuilder/myclasses folder. Find ApOut.html. If you ran the above program in Jbuilder, you can run it directly here by double-clicking on the ApOut.html file. Try it. It probably does NOT work, and will give you a message that it cannot find the file. This is because your browser has the Java virtual machine to run Java 1.1 applets but not Java 2 applets. You can install a plug-in (see page 432 of your text), but we will not worry about this added complication. We will run 1.1 code. It is a fairly simple process to convert between the two. Java 1.1 is easier to write and run, so it will be our choice for now. Here is the code for the corresponding Java 1.1 code for the above Java 2 example:
import java.applet.Applet;
import java.awt.*;
public class ApOut extends Applet
{
TextField t1;
Button b1;
Label l1;
public void init()
{
setBackground(Color.green);
t1 = new TextField(10);
add(t1);
b1 = new Button("press me");
add(b1);
l1 = new Label("this is a label");
add(l1);
}
}
Images:
Copy a jpeg or gif file into the folder Jbuilder/myclasses. Rename this file myPic.jpg or myPic.gif, depending on its type. Now copy and run the following class:
import java.awt.*;
import java.applet.Applet;
public class ApOut extends Applet
{
Image pic;
public void init()
{
pic = getImage(getDocumentBase(),"myPic.JPG");
}
public void paint(Graphics g)
{
g.drawImage(pic,0,0,this);
}
}
If your picture is myPic.gif, then make the appropriate change in the line: pic = getImage(getDocumentBase(),"myPic.JPG"); Run the program. You should see the image in the browser. Try playing with the g.drawImage statement in paint. Change the 0,0 to, say 30,30. Add this line to paint: g.drawImage(pic,0,120,getWidth(),getHeight()-120,this); Again, experiment!!
Putting it all together:
Your assignment is to use the classes and methods described above to make a full-page java applet with ‘interesting’ output. It does NOT have to look nice. It is more important to try lots of things. If you can make it look nice, then do so. You will add the code and output of this program to your Portfolio.
Bonus:
Copy and paste this code to ApOut:
import java.applet.*;
import java.awt.*;
public class ApOut extends Applet
{
int last_x, last_y; // Store the last mouse position.
Color current_color = Color.black; // Store the current color.
public void paint(Graphics g)
{
}
// This method is called when the user clicks the mouse to start a scribble.
public boolean mouseDown(Event e, int x, int y)
{
last_x = x; last_y = y;
return true;
}
// This method is called when the user drags the mouse.
public boolean mouseDrag(Event e, int x, int y)
{
Graphics g = this.getGraphics();
g.setColor(current_color);
g.drawLine(last_x, last_y, x, y);
last_x = x;
last_y = y;
return true;
}
// This method is called when the user clicks the button public boolean action(Event event, Object arg)
{
return super.action(event, arg);
}
} // end of class
Run this program. You should be able to draw on the browser as you click and drag the mouse. Look at the code and fiddle. What do you think each part does? Can you change the color of the drawing? See if you can draw on an image by adding an Image object, adding init and changing paint. In mouseDrag, try changing the drawLine method to the drawOval method for a wider paintbrush.
So.. by now you are probably exhausted and may want a diversion at my expense. Here you go..
|